Dev C++ Const
Constants are the data items that never change their value during a program run.
The xyz placement in the Scatter Matrix. will be returned by the 3 element array 'Order', where the index. of Order indicates the column (and row) in 'ScatterMatrix', and. a value of 0 means x, 1 means y, and 2 means z./ void FindScatterMatrix( const Vector &Points, const Vec3f &Centroid, float ScatterMatrix33, int Order3. LRESULT DispatchMessage( const MSG.lpMsg ); Parameters. Type: const MSG. A pointer to a structure that contains the message. The return value specifies the value returned by the window procedure. Although its meaning depends on the message being dispatched, the return value generally is ignored.
C++ Constants Example
Following is an example program of C++ constants :
When the above C++ Program is compile and executed, it will produce the following output:
C++ allows the following kinds of literals :
- integer-constant
- character-constant
- floating-constant
- string-literal
C++ Integer Constants
Integer constants are whole numbers without any fractional part.
Method to write integer constant is - An integer constant must have at least one digit and must not contain any decimal point. It may contain either + or - sign. A number with no sign is assumed to be positive. Commas cannot appear in an integer constant.
C++ allows the following three types of integer constants :
- Decimal ( base 10 )
- Octal ( base 8 )
- Hexadecimal ( base 16 )
Let's discuss all the three types one by one.
C++ Decimal Integer Constants
An integer constant consisting of a sequence of digits is taken to be decimal integer constant unless it begins with 0 ( digit zero ). For instance, 1234, 43, +85, -25 are decimal integer constants
C++ Decimal Integer Constants Example
Following is the example program of C++ decimal integer constants :
When the above C++ program is compile and executed, it will produce the following output:
C++ Octal Integer Constants
A sequence of digits starting with 0 ( digit zero ) is taken to be an octal integer. For instance, decimal integer 8 will be written as 010 as octal integer. (... 810 = 108) and decimal integer 12 will be written as 014 as octal integer (...1210 = 148)
The example program for C++ octal integer constants is same as above, but the number in this case is in octal form.
To convert a number from any number system to octal number system and vice-versa check the following:
C++ Hexadecimal Integer Constants
A sequence of digits preceded by 0x or 0X is taken to be an hexadecimal integer. For instance, decimal 12 will be written as 0XC as hexadecimal integer.
Thus number 12 will be written either as 12 ( as decimal ), 14 ( as octal ) and 0XC ( as hexadecimal ).
The suffix l or L and u or U attached to any constant forces it to be represented as a long and unsigned respectively.
The example program for C++ hexadecimal integer constants is same as above, but the number in this case is in hexadecimal form.
To convert a number from any number system to hexadecimal number system and vice-versa check the following:
C++ Character Constants
A character constant is one character enclosed in single quotes, as in 'z'.
The rule to write character constant is - A character constant in C++ must contain one character and must be enclosed in single quotation mark.
C++ Escape Sequences
C++ allows some non-graphic characters in character constant. Nongraphic characters are those characters that cannot be typed directly from keyboard. For example, backspace, tabs, carriage return etc. These nongraphic characters can be represented by using escape sequences. An escape sequence is represented by a backslash () followed by one or more characters. Following table lists the C++ Escape Sequences :
Escape Sequence | Nongraphic Character |
---|---|
a | Audible bell (alert) |
b | Backspace |
f | Formfeed |
n | Newline or linefeed |
r | Carriage Return |
t | Horizontal tab |
v | Vertical tab |
Backslash | |
' | Single quote |
' | Double quote |
? | Question mark |
On | Octal number (On represents the number in octal) |
xHn | Hexadecimal number (Hn represents the number in hexadecimal) |
0 | Null |
Note : An escape sequence represents a single character and hence consumes one byte in ASCII representation.
C++ Escape Sequences Example
Following is the example program of C++ escape sequence :
When the above program is compile and executed, it will produce the following output:
Floating Constants
Floating constants are also called as real constants.
Real constants are numbers having fractional parts. These may be written in one of the two forms called fractional form or the exponential form.
Dev C++ Console
A real constant in fraction form consists of signed or unsigned digits including a decimal point between digits.
The rule for writing a real constant in fractional form is - A real constant in fractional form must have at least one digit before a decimal point and at least one digit after the decimal point. It may also have either + or - sign preceding it. A real constant with no sign is assumed to be positive.
Following are some valid real constants in fractional form :
Following are some invalid real constants :
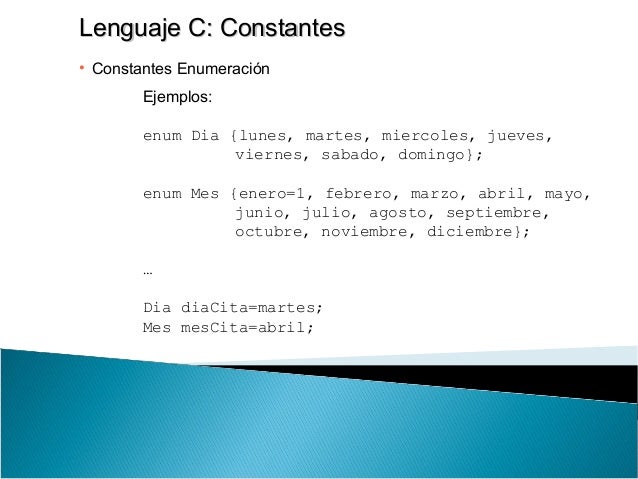
A real constant in exponent form consists of two parts : mantissa and exponent. For instance, 5.8 can be written as 0.58 * 101 = 0.58 E01 where mantissa part is 0.58 (the part appearing before E) and exponent part is 1 (the part appearing after E). E01 represents 101.
The rule for writing a real constant in exponent form is - A real constant is exponent form has two parts : a mantissa and an exponent. The mantissa must be either an integer or a proper real constant. The mantissa is followed by a letter E or e and the exponent. The exponent must be an integer.
Following are some valid real constants in exponent form :
Following are some invalid real constants in exponent form:
String Constants (Literals)
'Multiple Character' constants are treated as string-literals.
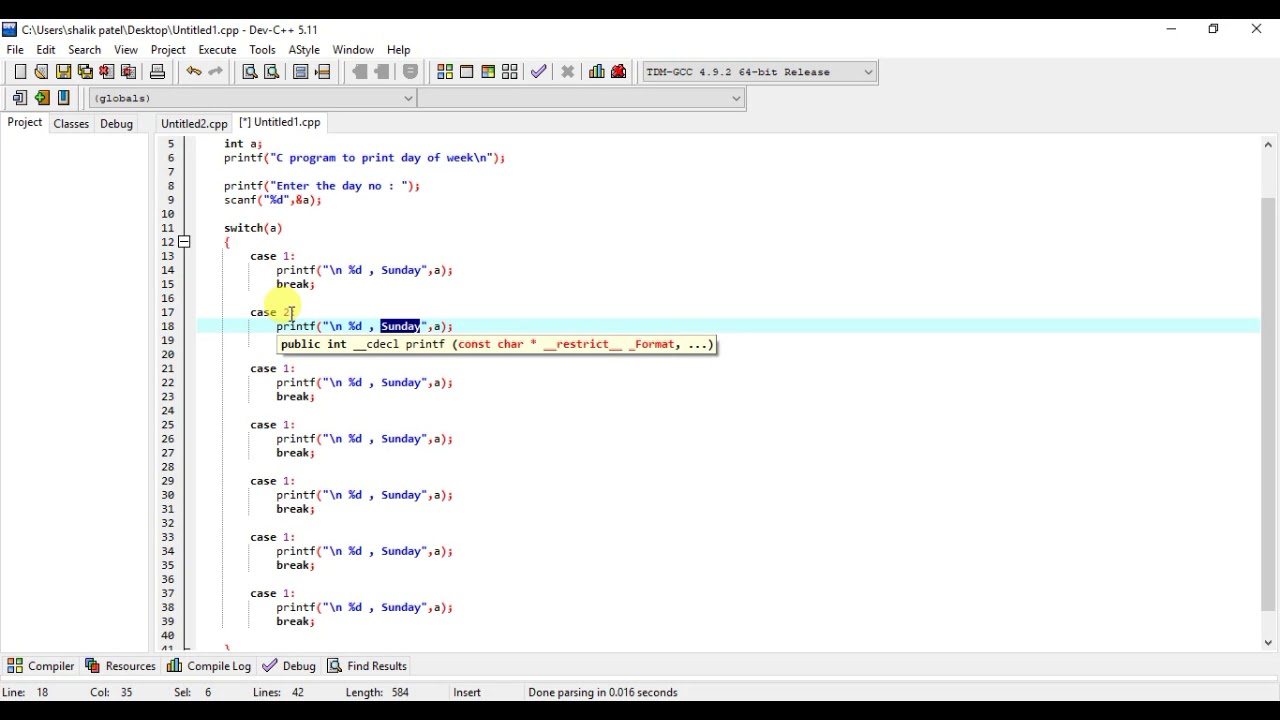
The rule to write string-literal is - A string literal is a sequence of characters surrounded by double quotes.
The difference between multicharacter character constants (which are generally used for writing octal numbers or hexadecimal numbers or ASCII numbers) and string-literals is that multicharacer character constants are treated as integers (int type) and their values are implementation (version) dependent. Whereas a string-literal is treated as array of char. The benefit of array is that each constituent character of the string can be dealt with separately.
Each string literal is by default (automatically) added with a special character '0' which makes the end of a string. '0' is a single character. Thus the size of a string is the number of characters plus one for this terminator. For example,
'abc' size is 4.
'ab' size is 3 (a is an escape sequence, thus one character).
'Subhu's pen' size is 12 (For typing apostrophe(') sign escape sequence has been used).
Thus 'abc' will actually be represented as 'abc0' in the memory i.e., along with the terminator character.
-->
Dispatches a message to a window procedure. It is typically used to dispatch a message retrieved by the GetMessage function.
Syntax
Parameters
lpMsg
Type: const MSG*
A pointer to a structure that contains the message.
Return value
Type: LRESULT
The return value specifies the value returned by the window procedure. Although its meaning depends on the message being dispatched, the return value generally is ignored.
Remarks
The MSG structure must contain valid message values. If the lpmsg parameter points to a WM_TIMER message and the lParam parameter of the WM_TIMER message is not NULL, lParam points to a function that is called instead of the window procedure.
Note that the application is responsible for retrieving and dispatching input messages to the dialog box. Most applications use the main message loop for this. However, to permit the user to move to and to select controls by using the keyboard, the application must call IsDialogMessage. For more information, see Dialog Box Keyboard Interface.
Examples
For an example, see Creating a Message Loop.
Requirements
Dev C++ Construction
Minimum supported client | Windows 2000 Professional [desktop apps only] |
Minimum supported server | Windows 2000 Server [desktop apps only] |
Target Platform | Windows |
Header | winuser.h (include Windows.h) |
Library | User32.lib |
DLL | User32.dll |
API set | ext-ms-win-ntuser-message-l1-1-0 (introduced in Windows 8) |
See also
Conceptual
Reference